The function principle of object-orientation is a foundational idea in software design. It helps us build systems that reflect how real-world agents interact. Instead of focusing on isolated tasks or raw data, object-oriented design organizes behavior, state, and communication into encapsulated units called objects.
This principle enables a structure where parts of a system act autonomously, send messages, and react to requests—just like actors in a real-world process. By modeling systems in this way, we gain clarity, modularity, and maintainability.
To make this more tangible, I’ll use an analogy: opening a stock portfolio at a bank and requesting to buy a share. This familiar scenario helps us explore the abstract concepts at play, without losing sight of the deeper principles.
What is Object Orientation?
Object orientation is a way to construct software by combining state, behavior, and communication into reusable components known as objects. Each object represents a small piece of the system. It knows things (data), it can do things (methods), and it communicates through messages.
At the heart of this model lies the function principle of object-orientation. According to this principle, every interaction is carried out through message passing between objects. The sender does not know how the receiver fulfills the request. It only knows what the receiver can do—not how it does it.
This encapsulation of functionality ensures that systems remain flexible and extendable over time. In other words, objects cooperate while respecting each other’s independence.
The Function Principle in Action: A Real-World Parallel
To make the function principle of object-orientation more accessible, let’s look at a common scenario.
I walk into a bank, I want to open a stock portfolio and purchase a share, and I speak to a bank employee, who takes care of both. They ask for my information, verify my identity, open the portfolio, and carry out the share purchase.
This simple interaction reflects the function principle precisely:
- I, the initiating object, send a message.
- The bank employee, another object, interprets and acts on that message.
- Internally, they may send further messages to other systems (e.g., a trading platform).
- None of this is visible to me—the initiating object.
This is the essence of object-oriented design: collaborating objects responding to requests, hiding their internal implementation while offering defined behaviors.
Thinking in Objects: Encapsulation and Communication
Let’s abstract this example.
Each participant in the process—customer, bank employee, trading system—is an object. Each has:
- A state (e.g., the customer’s personal data),
- Behaviors (e.g., openPortfolio, buyShare),
- And a public interface through which it communicates.
The customer object doesn’t know what’s happening inside the employee object. Similarly, the employee object doesn’t care whether the data is memorized or pulled from an ID. The only concern is: Is the request valid? Is it actionable?
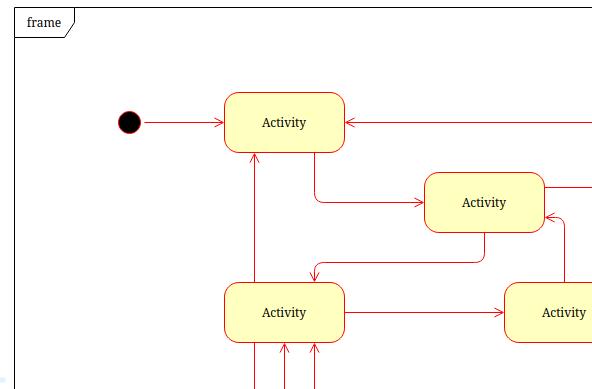
This leads us to an essential aspect of the function principle of object-orientation: encapsulation. Every object hides its inner structure and only exposes what other objects need to interact with it. This is also known as information hiding.
Message Passing: From Task to Chain
Under the function principle, an object:
- Sends a message (request) to another object.
- That object may then activate internal behavior or pass the task along.
- A chain of activations can form, where each object only knows its direct partner.
Returning to the analogy:
- I request a stock purchase.
- The bank employee forwards that to an investment system.
- That system calls a pricing service.
- The pricing service may query a data provider.
- And so on.
From my perspective, it’s just one request. But in software, it’s a chain of behavior activations, each isolated yet connected.
The Role of the Interface: Clarity and Independence
In object orientation, what matters is what an object offers, not how it offers it. This means that:
- An object must clearly define what services it provides.
- It must also protect how these services are internally implemented.
The customer knows the employee can “open a stock portfolio” and “buy a share.” But the employee might perform several checks, validations, and background processes. The interface abstracts this away, allowing smooth cooperation without unnecessary detail.
That’s exactly what the function principle of object-orientation promotes: independence through clear contracts and hidden complexity.
Mapping the Metaphor
Here’s how the real-world scenario maps to object-oriented concepts:
Real-World Element | Object-Oriented Equivalent |
---|---|
Customer | Object initiating the request |
Bank employee | Object receiving and processing |
Open stock portfolio | Method on bank object |
Buy a share | Another method (behavior) |
Personal data | Object attributes (internal state) |
Task request | Message to activate behavior |
Internal process | Hidden logic (encapsulation) |
Third-party systems | Collaborating internal objects |
The clarity of this mapping shows why the function principle is so central: it models relationships and cooperation, not just computation.
Chains of Delegation: Autonomous Execution
Here’s another layer of abstraction. The function principle assumes that once a task is assigned, the receiving object becomes fully responsible for completing it. It may:
- Ask other objects for help.
- Use its own state and methods.
- Or make autonomous decisions.
But crucially, the original sender doesn’t interfere.
In code, this reduces dependencies. And in design, it improves flexibility. And in teams, it encourages responsibility delegation. Once you understand this principle, you can build entire systems by simply defining roles and allowing them to interact.
Final Thoughts
The function principle of object-orientation is not just a technique—it’s a philosophy. It teaches us to build systems based on communication, autonomy, and abstraction. Each object becomes a small agent, each one has a purpose, and each one collaborates by contract, not by force.
This makes systems easier to build, easier to scale, and much easier to change.
When I walk into a bank, open a stock portfolio, and buy a share, I’m not just performing a financial transaction. I’m watching object-orientation in action. Every actor hides complexity, every message triggers behavior, and every behavior respects boundaries.
And that’s what makes this principle so enduring, so useful, and so powerful in the world of software.